Time value of money models¶
Overview¶
The functions in this module are used for certain compound interest calculations for a cashflow under the following restrictions:
- Payment periods coincide with the compounding periods.
- Payments occur at regular intervals.
- Payments are a constant amount.
- Interest rate is the same over all analysis period.
For convenience of the user, this module implements the following simplified functions:
pvfv
: computes the missing value in the equationfval = pval * (1 + rate) ** nper
, that represents the following cashflow.
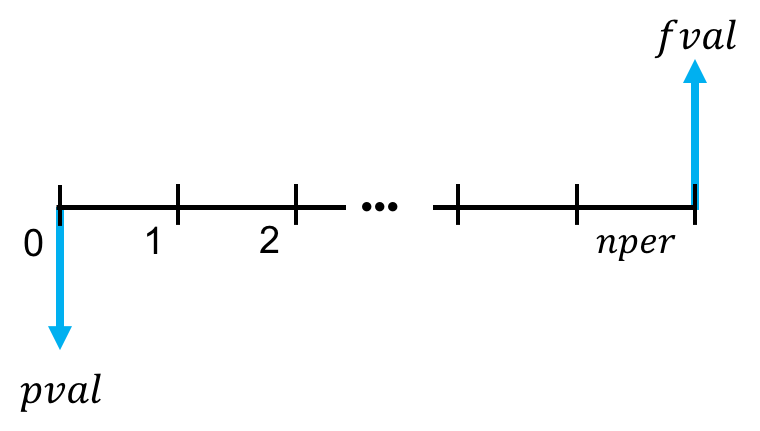
pvpmt
: computes the missing value (pmt, pval, nper, nrate) in a model relating a present value and a finite sequence of payments made at the end of the period (payments in arrears, or ordinary annuities), as indicated in the following cashflow diagram:
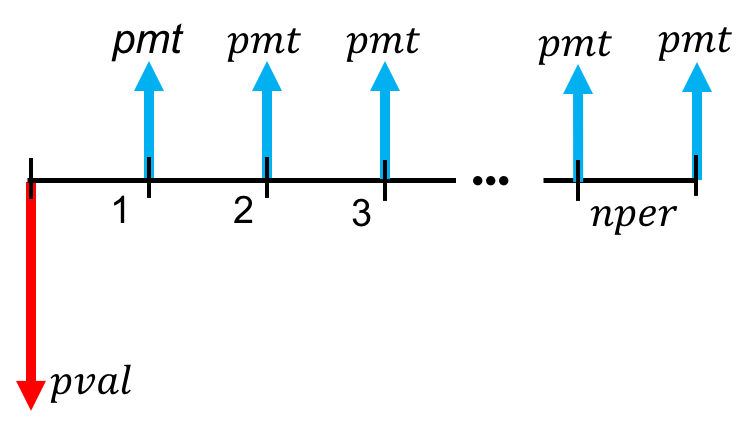
pmtfv
: computes the missing value (pmt, fval, nper, nrate) in a model relating a finite sequence of payments in advance (annuities due) and a future value, as indicated in the following diagram:
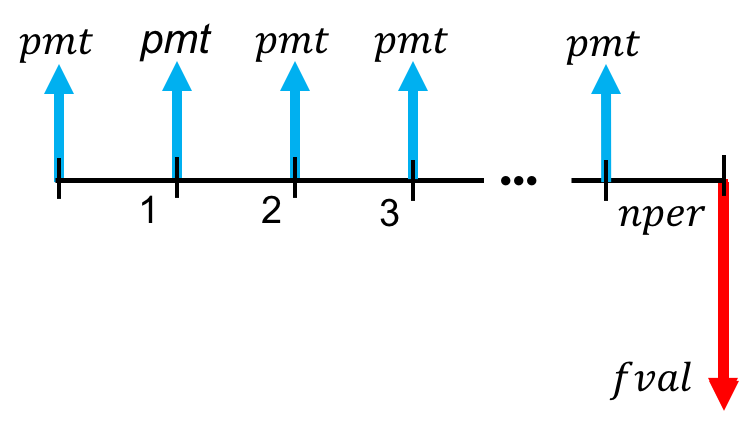
tvmm
: computes the missing value (pmt, fval, pval, `nper, nrate) in a model relating a finite sequence of payments made at the beginning or at the end of the period, a present value, a future value, and an interest rate, as indicated in the following diagram:
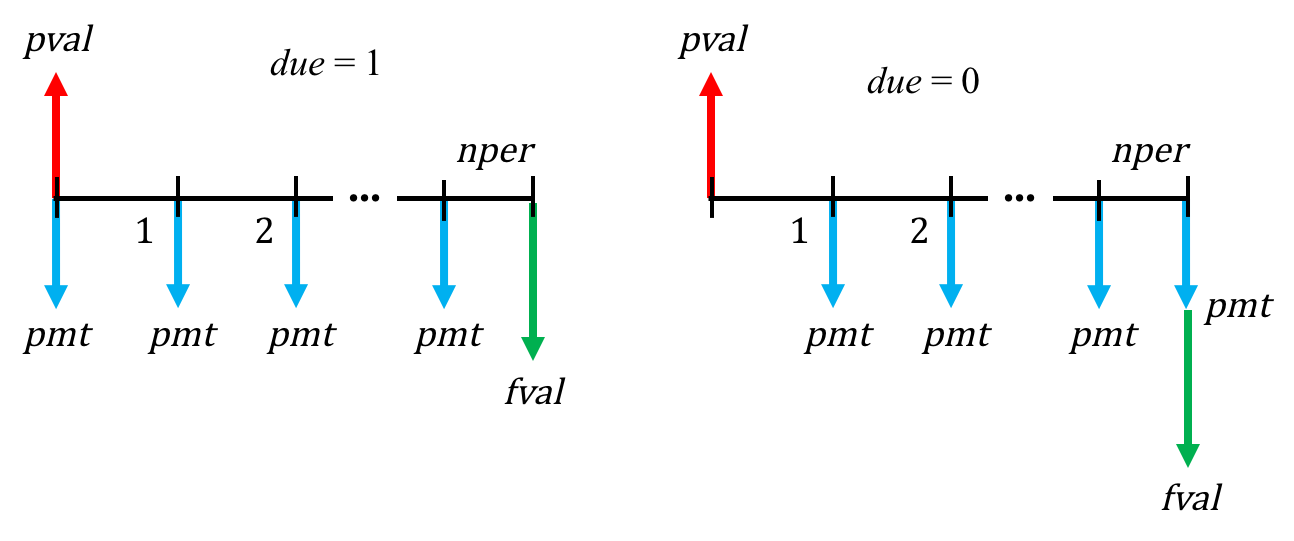
In addition, the function amortize
computes and returns the amortization
schedule of a loan.
Functions in this module¶
-
cashflows.tvmm.
amortize
(pval=None, fval=None, pmt=None, nrate=None, nper=None, due=0, pyr=1, noprint=True)[source]¶ Amortization schedule of a loan.
Parameters: - pval (float) – present value.
- fval (float) – Future value.
- pmt (float) – periodic payment per period.
- nrate (float) – nominal interest rate per year.
- nper (int) – total number of compounding periods.
- due (int) – When payments are due.
- pyr (int, list) – number of periods per year.
- noprint (bool) – prints enhanced output
Returns: (principal, interest, payment, balance)
Return type: A tuple
Details
The
amortize
function computes and returns the columns of a amortization schedule of a loan. The function returns the interest payment, the principal repayment, the periodic payment and the balance at the end of each period.Examples
The amortization schedule for a loan of 100, at a interest rate of 10%, and a life of 5 periods, can be computed as:
>>> pmt = tvmm(pval=100, nrate=10, nper=5, fval=0) >>> amortize(pval=100, nrate=10, nper=5, fval=0, noprint=False) t Beginning Periodic Interest Principal Final Principal Payment Payment Repayment Principal Amount Amount Amount -------------------------------------------------------------------- 0 100.00 0.00 0.00 0.00 100.00 1 100.00 -26.38 10.00 -16.38 83.62 2 83.62 -26.38 8.36 -18.02 65.60 3 65.60 -26.38 6.56 -19.82 45.78 4 45.78 -26.38 4.58 -21.80 23.98 5 23.98 -26.38 2.40 -23.98 0.00
>>> amortize(pval=-100, nrate=10, nper=5, fval=0, noprint=False) t Beginning Periodic Interest Principal Final Principal Payment Payment Repayment Principal Amount Amount Amount -------------------------------------------------------------------- 0 -100.00 0.00 0.00 0.00 -100.00 1 -100.00 26.38 -10.00 16.38 -83.62 2 -83.62 26.38 -8.36 18.02 -65.60 3 -65.60 26.38 -6.56 19.82 -45.78 4 -45.78 26.38 -4.58 21.80 -23.98 5 -23.98 26.38 -2.40 23.98 -0.00
In the next example, the argument
due
is used to indicate that the periodic payment occurs at the begining of period.>>> amortize(pval=100, nrate=10, nper=5, fval=0, due=1, noprint=False) t Beginning Periodic Interest Principal Final Principal Payment Payment Repayment Principal Amount Amount Amount -------------------------------------------------------------------- 0 100.00 -23.98 0.00 -23.98 76.02 1 76.02 -23.98 7.60 -16.38 59.64 2 59.64 -23.98 5.96 -18.02 41.62 3 41.62 -23.98 4.16 -19.82 21.80 4 21.80 -23.98 2.18 -21.80 0.00 5 0.00 0.00 0.00 0.00 0.00
>>> amortize(pval=-100, nrate=10, nper=5, fval=0, due=1, noprint=False) t Beginning Periodic Interest Principal Final Principal Payment Payment Repayment Principal Amount Amount Amount -------------------------------------------------------------------- 0 -100.00 23.98 0.00 23.98 -76.02 1 -76.02 23.98 -7.60 16.38 -59.64 2 -59.64 23.98 -5.96 18.02 -41.62 3 -41.62 23.98 -4.16 19.82 -21.80 4 -21.80 23.98 -2.18 21.80 -0.00 5 -0.00 0.00 -0.00 -0.00 -0.00
The function returns a tuple with the columns of the amortization schedule.
>>> principal, interest, payment, balance = amortize(pval=100, ... nrate=10, nper=5, fval=0)
>>> principal [0, -16.37..., -18.01..., -19.81..., -21.80..., -23.98...]
>>> interest [0, 10.0, 8.36..., 6.56..., 4.57..., 2.39...]
>>> payment [0, -26.37..., -26.37..., -26.37..., -26.37..., -26.37...]
>>> balance [100, 83.62..., 65.60..., 45.78..., 23.98..., 1...]
In the following examples, the
sum
function is used to sum of different columns of the amortization schedule.>>> principal, interest, payment, balance = amortize(pval=100, ... nrate=10, nper=5, pmt=pmt)
>>> sum(interest) 31.89...
>>> sum(principal) -99.99...
>>> principal, interest, payment, balance = amortize(fval=0, ... nrate=10, nper=5, pmt=pmt)
>>> sum(interest) 31.89...
>>> sum(principal) -99.99...
>>> principal, interest, payment, balance = amortize(pval=100, ... fval=0, nper=5, pmt=pmt)
>>> sum(interest) 31.89...
>>> sum(principal) -99.99...
>>> amortize(pval=100, fval=0, nrate=10, pmt=pmt, noprint=False) t Beginning Periodic Interest Principal Final Principal Payment Payment Repayment Principal Amount Amount Amount -------------------------------------------------------------------- 0 100.00 0.00 0.00 0.00 100.00 1 100.00 -26.38 10.00 -16.38 83.62 2 83.62 -26.38 8.36 -18.02 65.60 3 65.60 -26.38 6.56 -19.82 45.78 4 45.78 -26.38 4.58 -21.80 23.98 5 23.98 -26.38 2.40 -23.98 0.00
>>> principal, interest, payment, balance = amortize(pval=100, ... fval=0, nrate=10, pmt=pmt)
>>> sum(interest) 31.89...
>>> sum(principal) -99.99...
-
cashflows.tvmm.
pmtfv
(pmt=None, fval=None, nrate=None, nper=None, pyr=1, noprint=True)[source]¶ Computes the missing argument (set to
None
) in a model relating the the future value, the periodic payment, the number of compounding periods and the nominal interest rate in a cashflow.Parameters: - pmt (float, list) – Periodic payment.
- fval (float, list) – Future value.
- nrate (float, list) – Nominal rate per year.
- nper (int, list) – Number of compounding periods.
- pyr (int, list) – number of periods per year.
- noprint (bool) – prints enhanced output
Returns: The value of the parameter set to None in the function call.
Details
The
pmtfv
function computes and returns the missing value (pmt
,fval
,nper
,nrate
) in a model relating a finite sequence of payments made at the beginning or at the end of each period, a future value, and a nominal interest rate. The time intervals between consecutive payments are assumed to be equial. For internal computations, the effective interest rate per period is calculated asnrate / pyr
.This function is used to simplify the call to the
tvmm
function. See thetvmm
function for details.
-
cashflows.tvmm.
pvfv
(pval=None, fval=None, nrate=None, nper=None, pyr=1, noprint=True)[source]¶ Computes the missing argument (set to
None
) in a model relating the present value, the future value, the number of compoundig periods and the nominal interest rate in a cashflow.Parameters: - pval (float, list) – Present value.
- fval (float, list) – Future value.
- nrate (float, list) – Nominal interest rate per year.
- nper (int, list) – Number of compounding periods.
- pyr (int, list) – number of periods per year.
- noprint (bool) – prints enhanced output
Returns: The value of the parameter set to
None
in the function call.Details
The
pvfv
function computes and returns the missing value (fval
,pval
,nper
,nrate
) in a model relating these variables. The time intervals between consecutive payments are assumed to be equial. For internal computations, the effective interest rate per period is calculated asnrate / pyr
.This function is used to simplify the call to the
tvmm
function. See thetvmm
function for details.
-
cashflows.tvmm.
pvpmt
(pmt=None, pval=None, nrate=None, nper=None, pyr=1, noprint=True)[source]¶ Computes the missing argument (set to
None
) in a model relating the present value, the periodic payment, the number of compounding periods and the nominal interest rate in a cashflow.Parameters: - pmt (float, list) – Periodic payment.
- pval (float, list) – Present value.
- nrate (float, list) – Nominal interest rate per year.
- nper (int, list) – Number of compounding periods.
- pyr (int, list) – number of periods per year.
- noprint (bool) – prints enhanced output
Returns: The value of the parameter set to None in the function call.
Details
The
pvpmt
function computes and returns the missing value (pmt
,pval
,nper
,nrate
) in a model relating a finite sequence of payments made at the beginning or at the end of each period, a present value, and a nominal interest rate. The time intervals between consecutive payments are assumed to be equial. For internal computations, the effective interest rate per period is calculated asnrate / pyr
.This function is used to simplify the call to the
tvmm
function. See thetvmm
function for details.
-
cashflows.tvmm.
tvmm
(pval=None, fval=None, pmt=None, nrate=None, nper=None, due=0, pyr=1, noprint=True)[source]¶ Computes the missing argument (set to
None
) in a model relating the present value, the future value, the periodic payment, the number of compounding periods and the nominal interest rate in a cashflow.Parameters: - pval (float, list) – Present value.
- fval (float, list) – Future value.
- pmt (float, list) – Periodic payment.
- nrate (float, list) – Nominal interest rate per year.
- nper (int, list) – Number of compounding periods.
- due (int) – When payments are due.
- pyr (int, list) – number of periods per year.
- noprint (bool) – prints enhanced output
Returns: Argument set to None in the function call.
Details
The
tvmmm
function computes and returns the missing value (pmt
,fval
,pval
,nper
,nrate
) in a model relating a finite sequence of payments made at the beginning or at the end of each period, a present value, a future value, and a nominal interest rate. The time intervals between consecutive payments are assumed to be equal. For internal computations, the effective interest rate per period is calculated asnrate / pyr
.Examples
This example shows how to find different values for a loan of 5000, with a monthly payment of 130 at the end of the month, a life of 48 periods, and a interest rate of 0.94 per month (equivalent to a nominal interest rate of 11.32%). For a loan, the future value is 0.
- Monthly payments: Note that the parameter
pmt
is set toNone
.
>>> tvmm(pval=5000, nrate=11.32, nper=48, fval=0, pyr=12) -130.00...
When the parameter
noprint
is set toFalse
, a user friendly table with the data computed by the function is print.>>> tvmm(pval=5000, nrate=11.32, nper=48, fval=0, pyr=12, noprint=False) Present Value: ....... 5000.00 Future Value: ........ 0.00 Payment: ............. -130.01 Due: ................. END No. of Periods: ...... 48.00 Compoundings per Year: 12 Nominal Rate: ....... 11.32 Effective Rate: ..... 11.93 Periodic Rate: ...... 0.94
- Future value:
>>> tvmm(pval=5000, nrate=11.32, nper=48, pmt=pmt, fval=None, pyr=12) -0.0...
- Present value:
>>> tvmm(nrate=11.32, nper=48, pmt=pmt, fval = 0.0, pval=None, pyr=12) 5000...
All the arguments support lists as inputs. When a argument is a list and the
noprint
is set toFalse
, a table with the data is print.>>> tvmm(pval=[5, 500, 5], nrate=11.32, nper=48, fval=0, pyr=12, noprint=False) # pval fval pmt nper nrate erate prate due ------------------------------------------------------ 0 5.00 0.00 -0.13 48.00 11.32 11.93 0.94 END 1 500.00 0.00 -0.13 48.00 11.32 11.93 0.94 END 2 5.00 0.00 -0.13 48.00 11.32 11.93 0.94 END
- Interest rate:
>>> tvmm(pval=5000, nper=48, pmt=pmt, fval = 0.0, pyr=12) 11.32...
- Number of periods:
>>> tvmm(pval=5000, nrate=11.32/12, pmt=pmt, fval=0.0) 48.0...